Overlay
Overlays parent element with div element with any color and opacity
Import
Polymorphic
Source
Docs
Package
Usage
Overlay
takes 100% of width and height of parent container or viewport if fixed
prop is set.
Set color
and backgroundOpacity
props to change Overlay
background-color. Note that backgroundOpacity
prop
does not change CSS opacity property, it changes background-color. For example, if you set
color="#000"
and backgroundOpacity={0.85}
background-color will be rgba(0, 0, 0, 0.85)
:
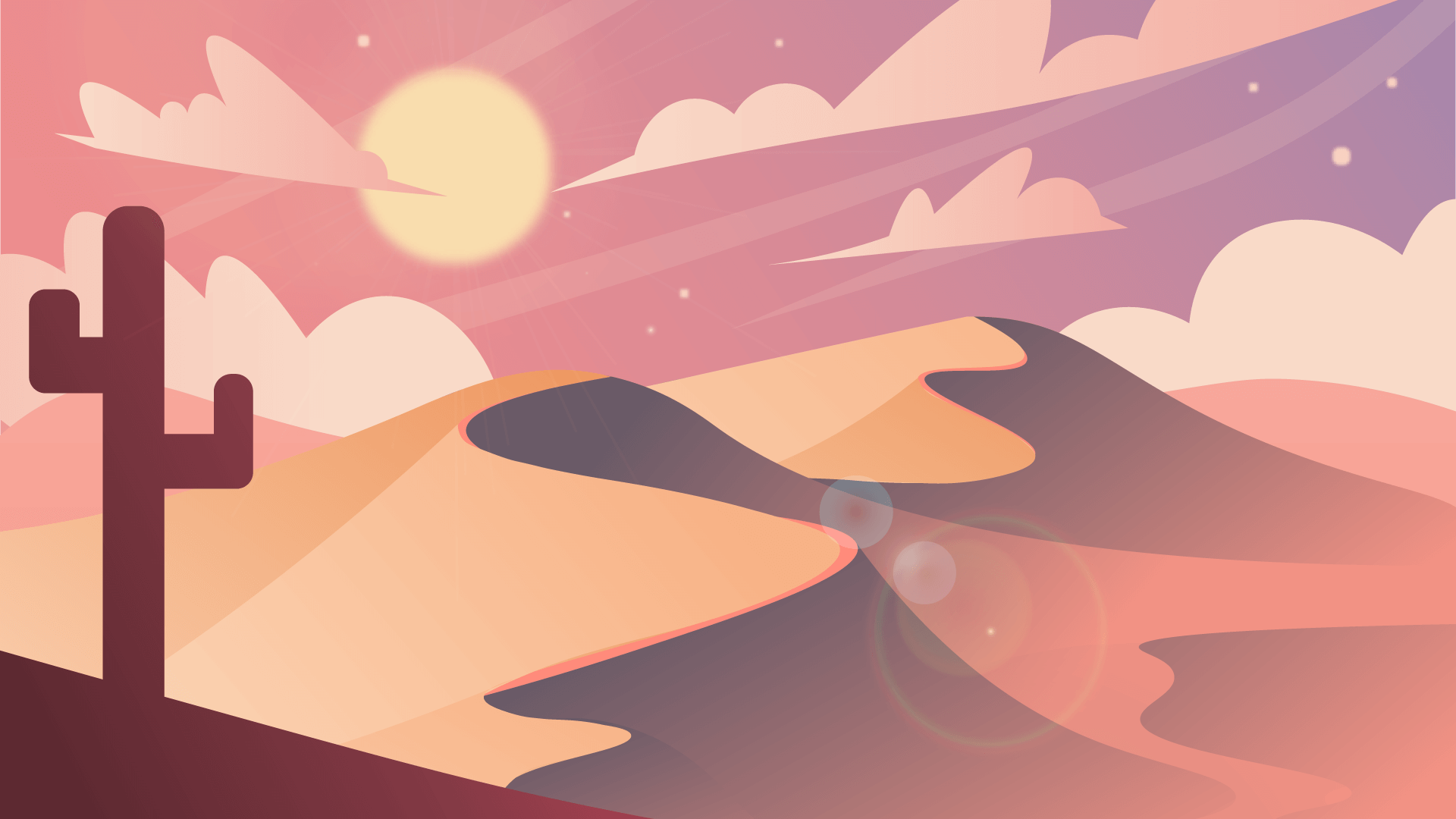
Gradient
Set gradient
prop to use background-image instead of background-color. When gradient
prop is set,
color
and backgroundOpacity
props are ignored.
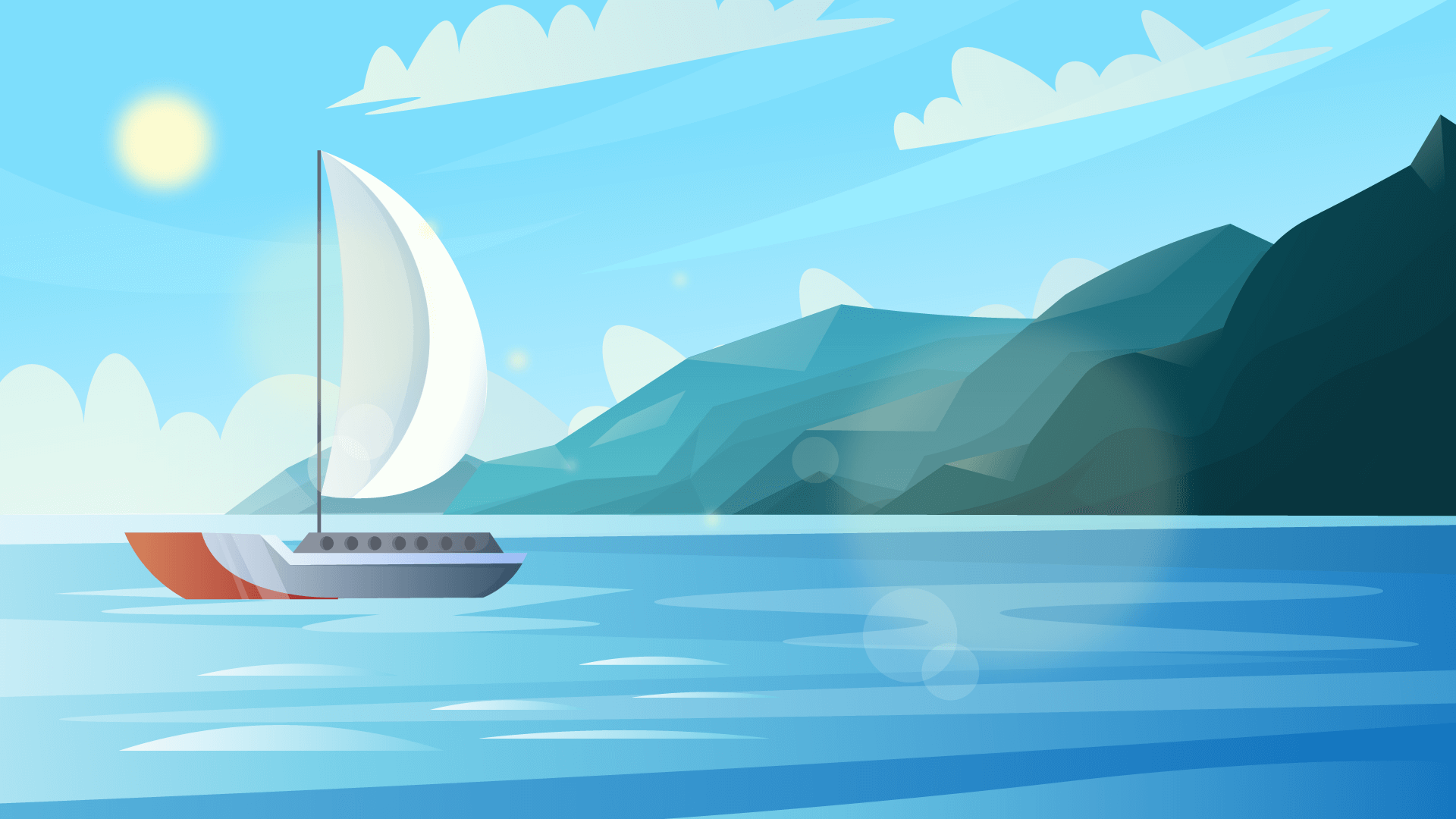
Blur
Set blur
prop to add backdrop-filter: blur({value})
styles.
Note that backdrop-filter
is not supported in all browsers.
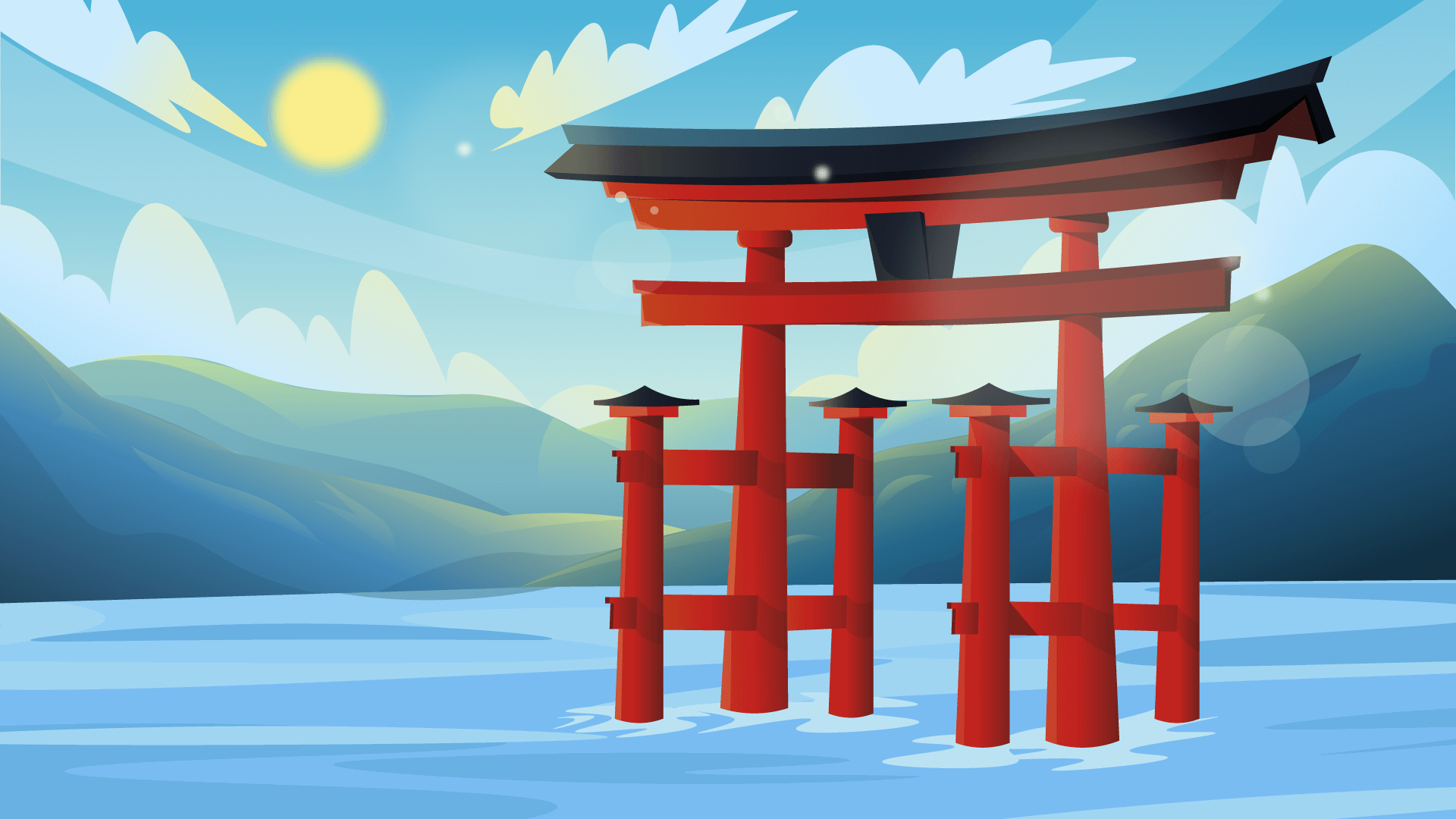
Polymorphic component
Overlay
is a polymorphic component – its default root element is div
, but it can be changed to any other element or component with component
prop:
You can also use components in component
prop, for example, Next.js Link
:
Polymorphic components with TypeScript
Note that polymorphic components props types are different from regular components – they do not extend HTML element props of the default element. For example,
OverlayProps
does not extendReact.ComponentPropsWithoutRef'<'div'>'
althoughdiv
is the default element.If you want to create a wrapper for a polymorphic component that is not polymorphic (does not support
component
prop), then your component props interface should extend HTML element props, for example:If you want your component to remain polymorphic after wrapping, use
createPolymorphicComponent
function described in this guide.