Indicator
Display element at the corner of another element
Import
Source
Docs
Package
Usage
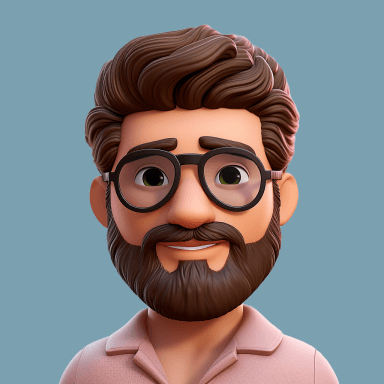
Color
Radius
Size
Inline
When the target element has a fixed width, set inline
prop to add display: inline-block;
styles to
Indicator container. Alternatively, you can set width and height with style
prop if you still want the root
element to keep display: block
.
New
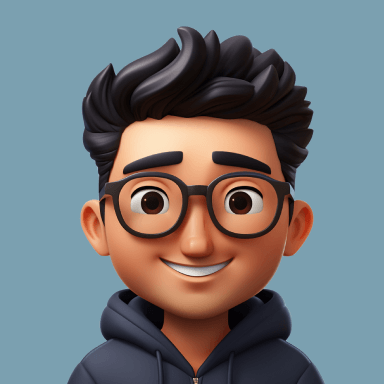
Offset
Set offset
to change indicator position. It is useful when Indicator component is
used with children that have border-radius:
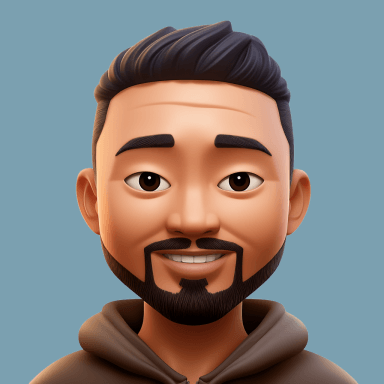
Processing animation
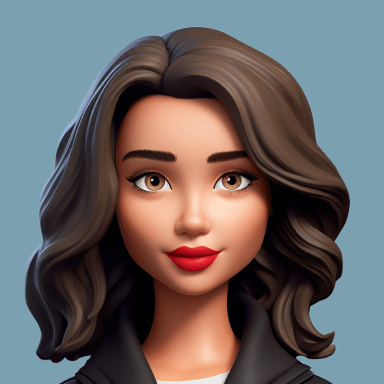
Disabled
Set disabled
to hide the indicator:
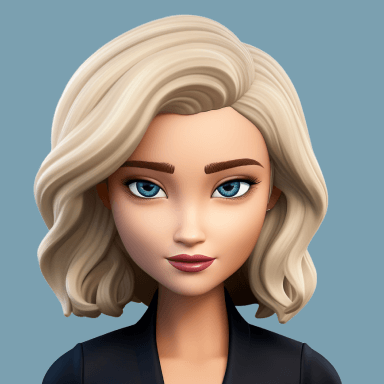