Image
Image with optional fallback
Import
Polymorphic
Source
Docs
Package
Usage
Image
is a wrapper for img
with minimal styles. By default, the image
will take 100% of parent width. The image size can be controlled with w
and h
style props.
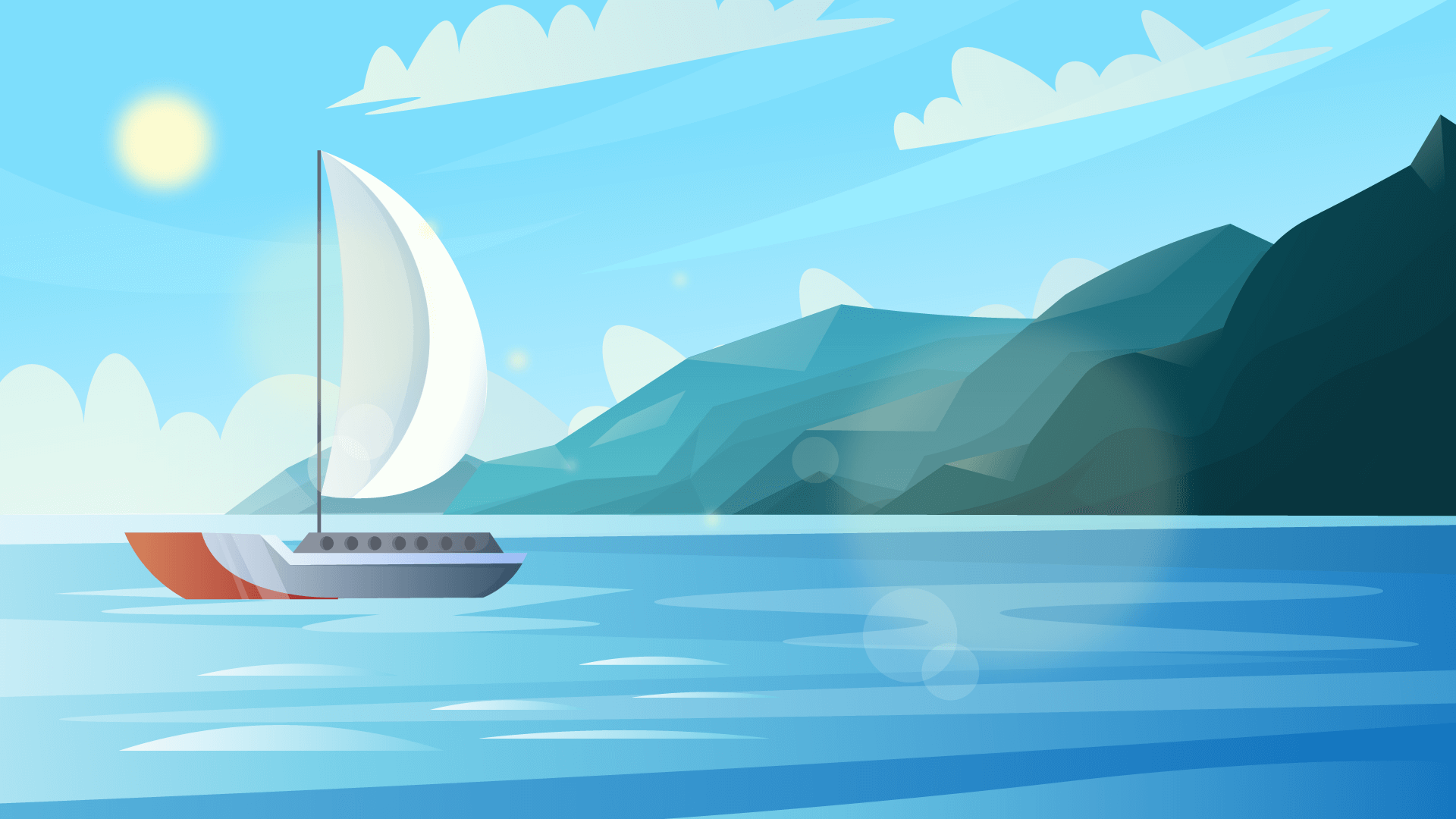
Image height
In most case, you will need to set image height to prevent layout jumps when
image is loading. You can do so with h
style props.
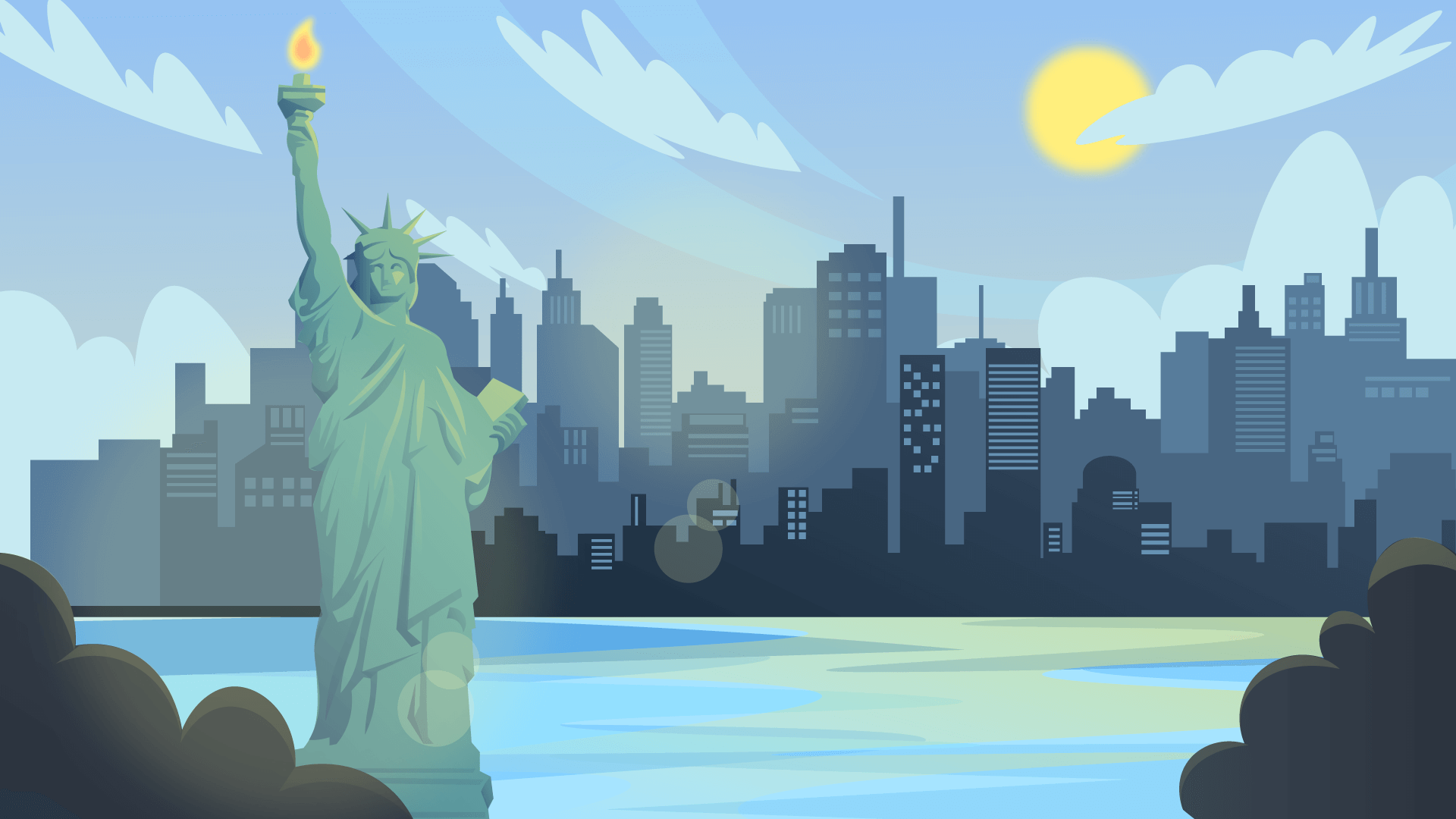
Image fit
By default the image has object-fit: cover
style - it will
resize to cover parent element. To change this behavior, set w="auto"
and fit="contain"
props.
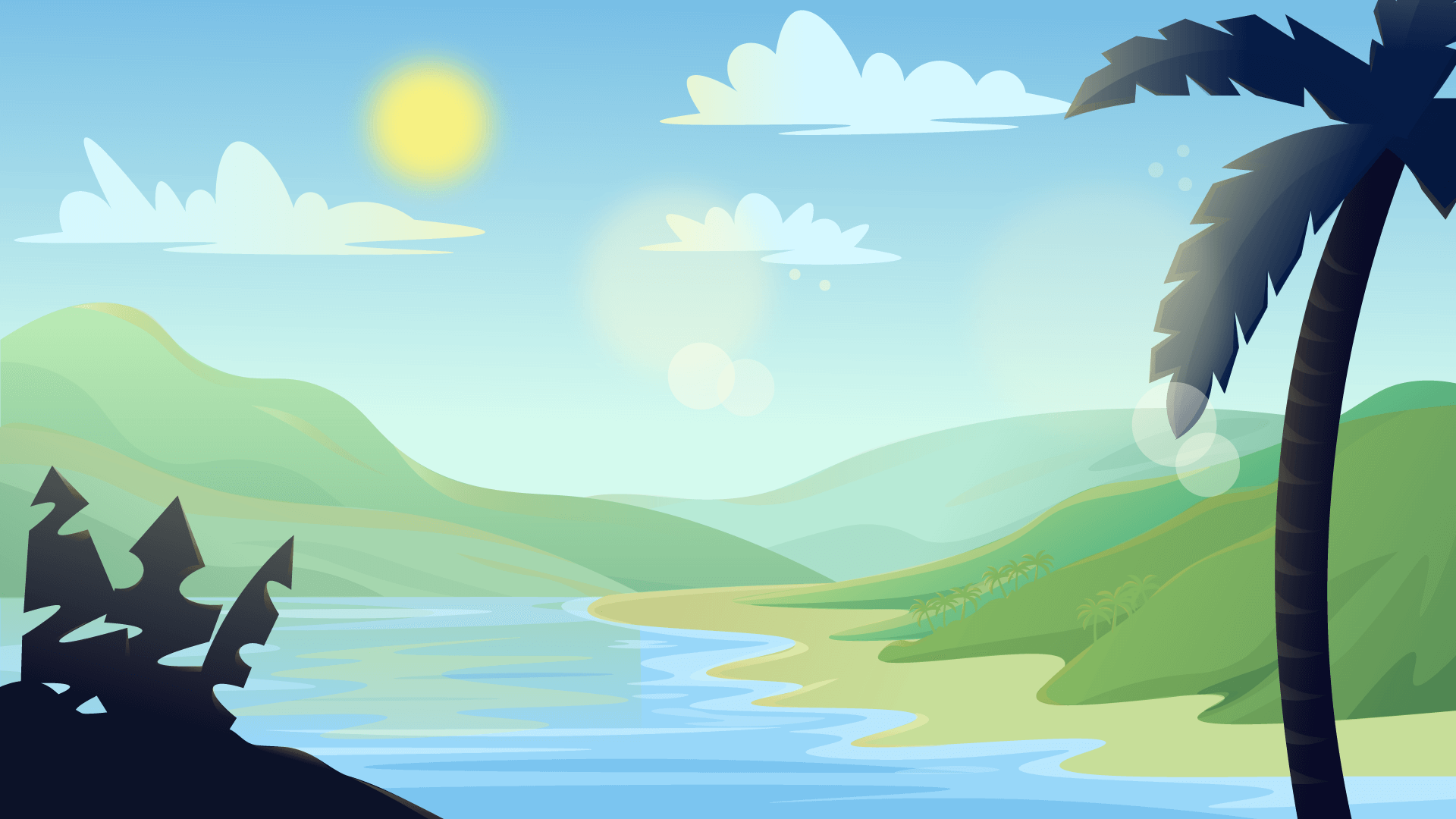
Fallback image
Set fallbackSrc
prop to display fallback image when image fails to load:
Usage with Next.js Image
Image
component is a polymorphic component, its root element can be changed with component
prop.
You can use it with next/image
and other similar components.