Avatar
Display user profile image, initials or fallback icon
Polymorphic
Source
Docs
Package
Usage
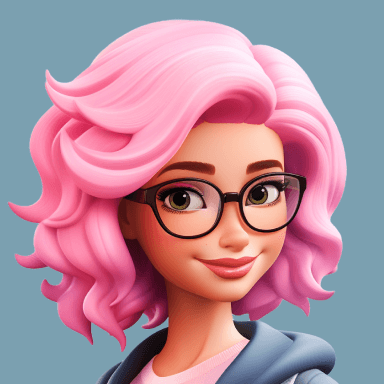
Initials
To display initials instead of the default placeholder, set name
prop
to the name of the person, for example, name="John Doe"
. If the name
is set, you can use color="initials"
to generate color based on the name:
Allowed initials colors
By default, all colors from the default theme are allowed for initials, you can restrict them
by providing allowedInitialsColors
prop with an array of colors. Note that the default colors
array does not include custom colors defined in the theme, you need to provide them manually
if needed.
Placeholder
If the image cannot be loaded or not provided, Avatar
will display a placeholder instead. By default,
placeholder is an icon, but it can be changed to any React node:
Variants
Avatar.Group
Avatar.Group
component combines multiple avatars into a stack:
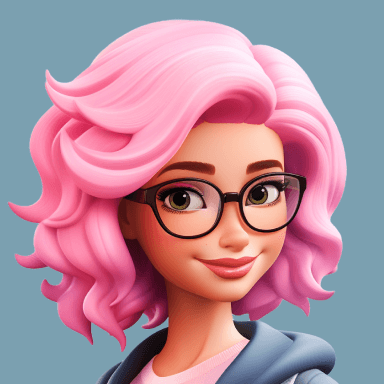
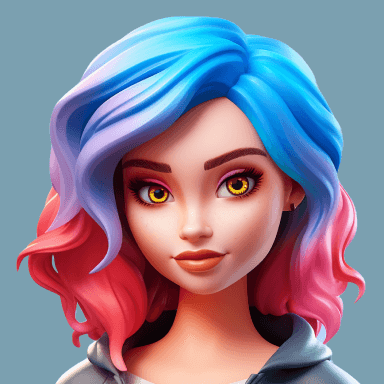
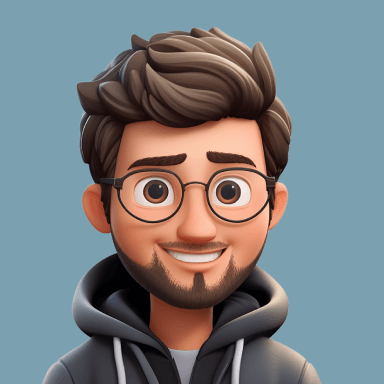
Note that you must not wrap child Avatar
components with any additional elements,
but you can use wrap Avatar
with components that do not render any HTML elements
in the current tree, for example Tooltip.
Example of usage with Tooltip:
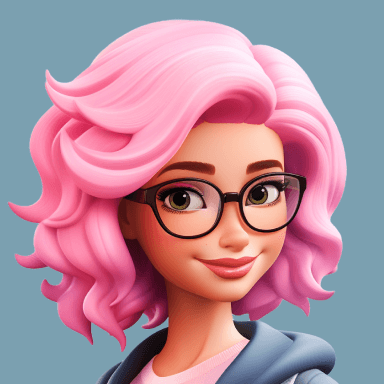
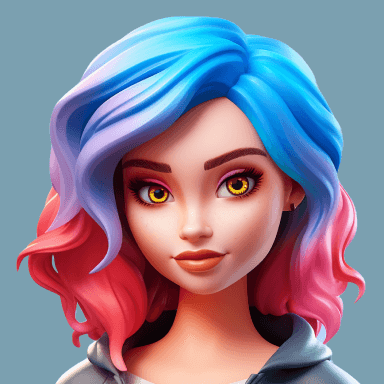
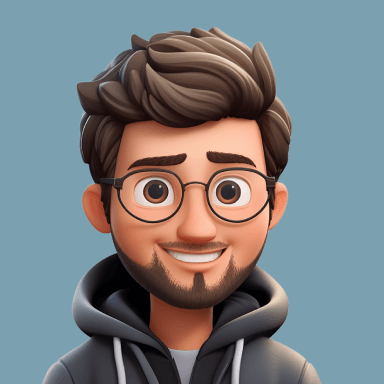
Polymorphic component
Avatar
is a polymorphic component – its default root element is div
, but it can be changed to any other element or component with component
prop:
You can also use components in component
prop, for example, Next.js Link
:
Polymorphic components with TypeScript
Note that polymorphic components props types are different from regular components – they do not extend HTML element props of the default element. For example,
AvatarProps
does not extendReact.ComponentPropsWithoutRef'<'div'>'
althoughdiv
is the default element.If you want to create a wrapper for a polymorphic component that is not polymorphic (does not support
component
prop), then your component props interface should extend HTML element props, for example:If you want your component to remain polymorphic after wrapping, use
createPolymorphicComponent
function described in this guide.
You can combine it with Tooltip or Popover to show additional information on hover
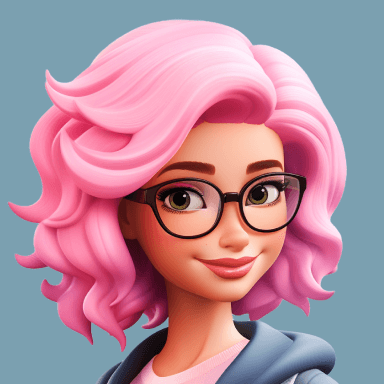
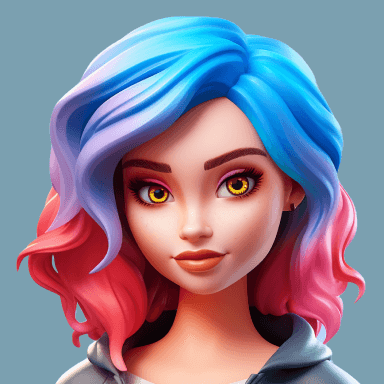
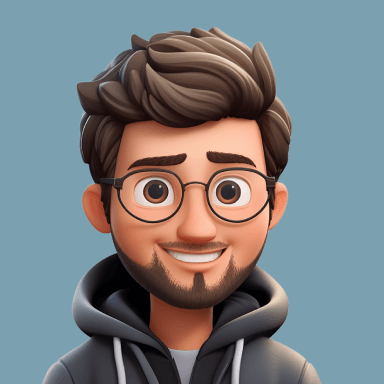
Accessibility
Avatar renders <img />
HTML element. Set alt
prop to describe image,
it is also used as title
attribute for avatar placeholder when the image cannot be loaded.