use-fullscreen
Enter/exit fullscreen mode with given element or entire page
Import
Source
Docs
Package
Usage
use-fullscreen
allows to enter/exit fullscreen for given element using the Fullscreen API.
By default, if you don't provide ref
, the hook will target document.documentElement
:
Custom target element
The hook returns an optional ref
function that can be passed to an element to act as root.
Be sure to follow best practices to not confuse or trap the end user:
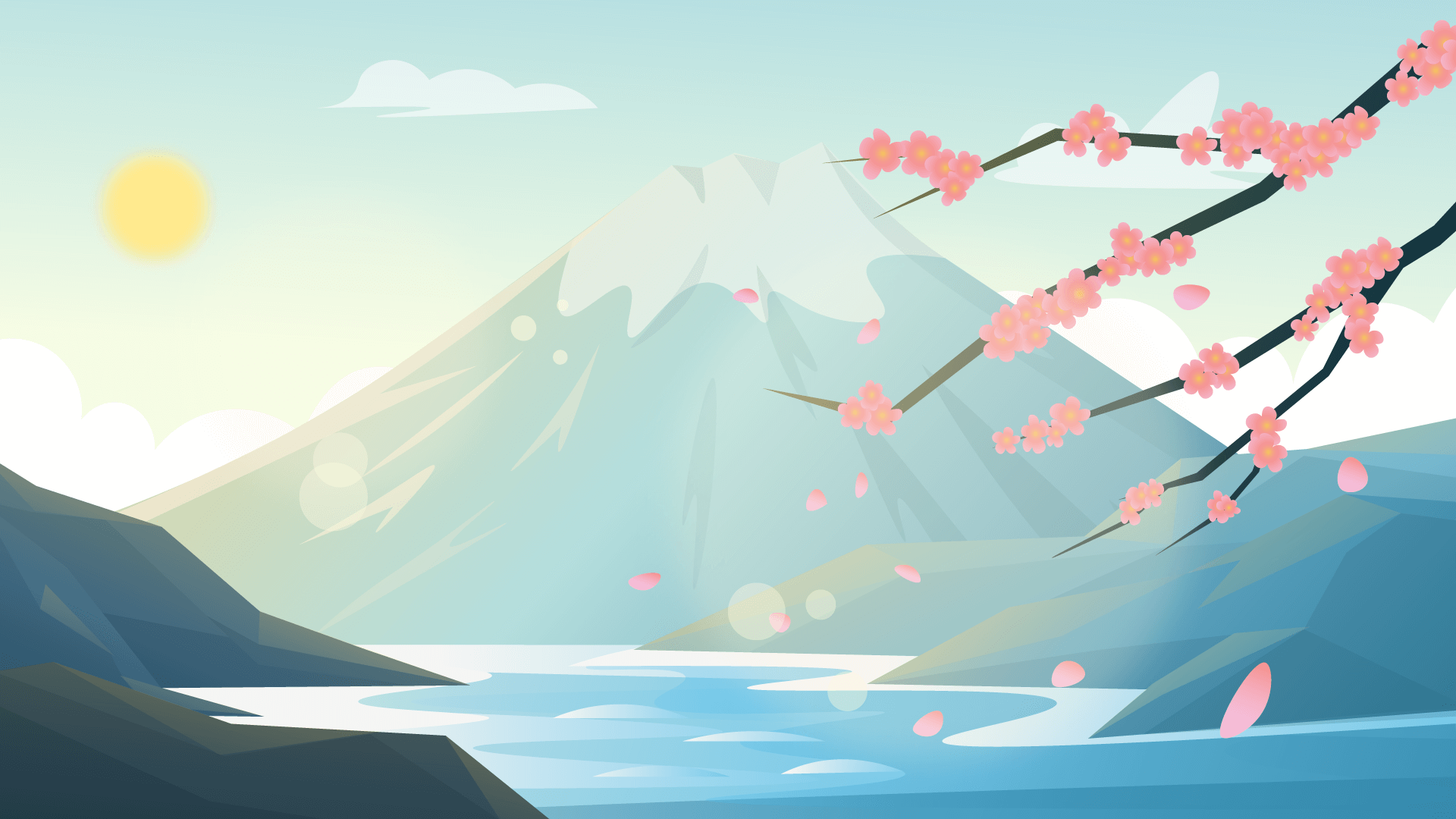